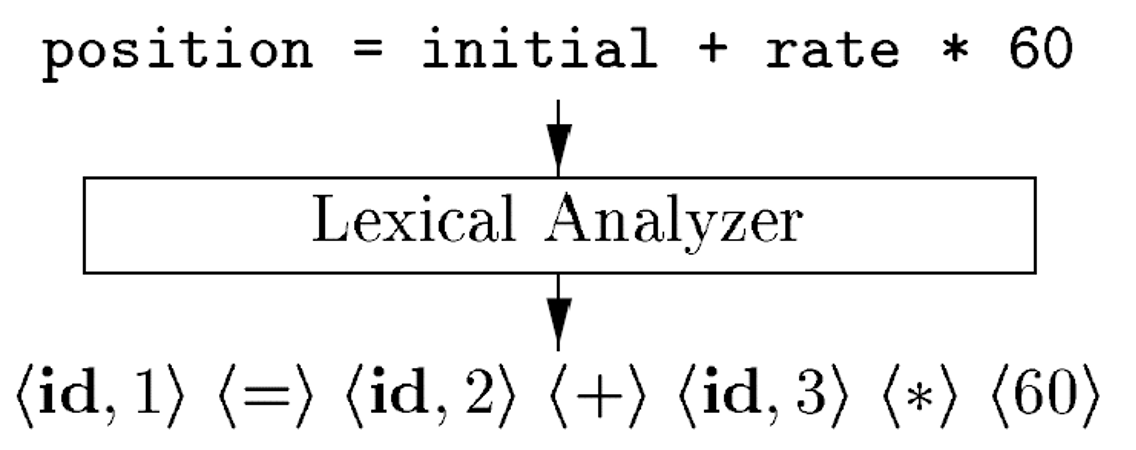
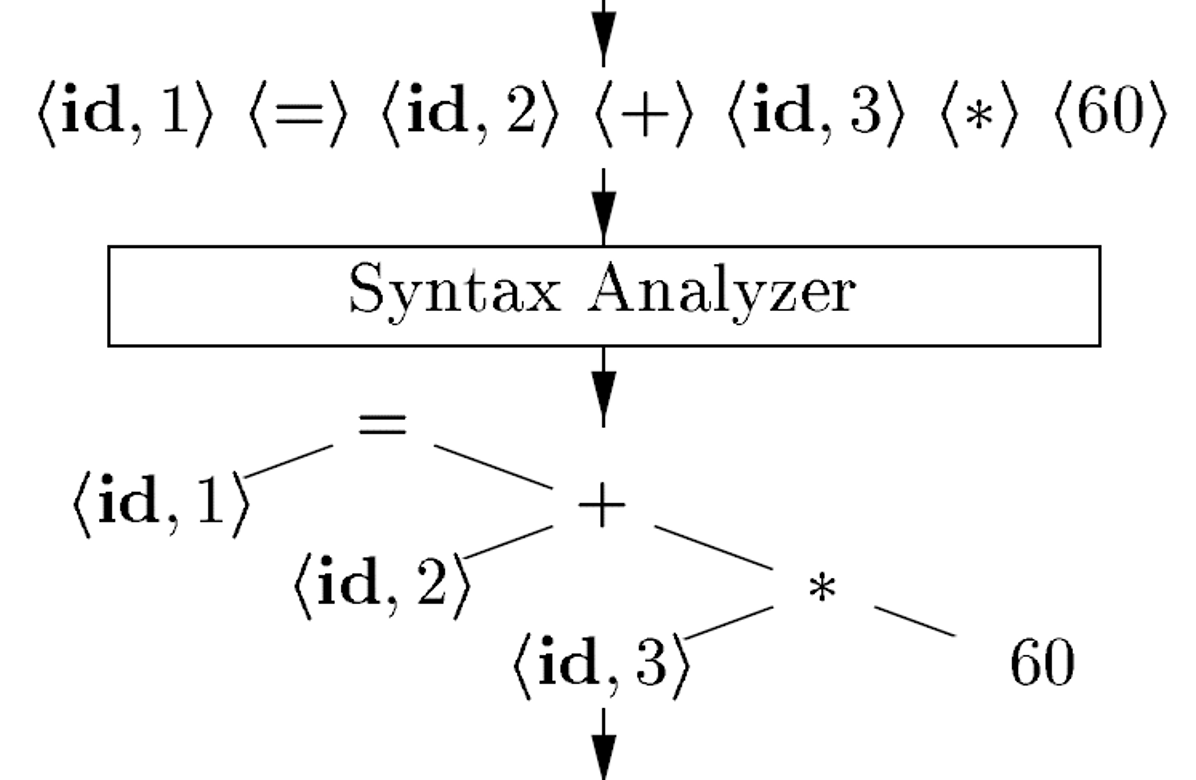
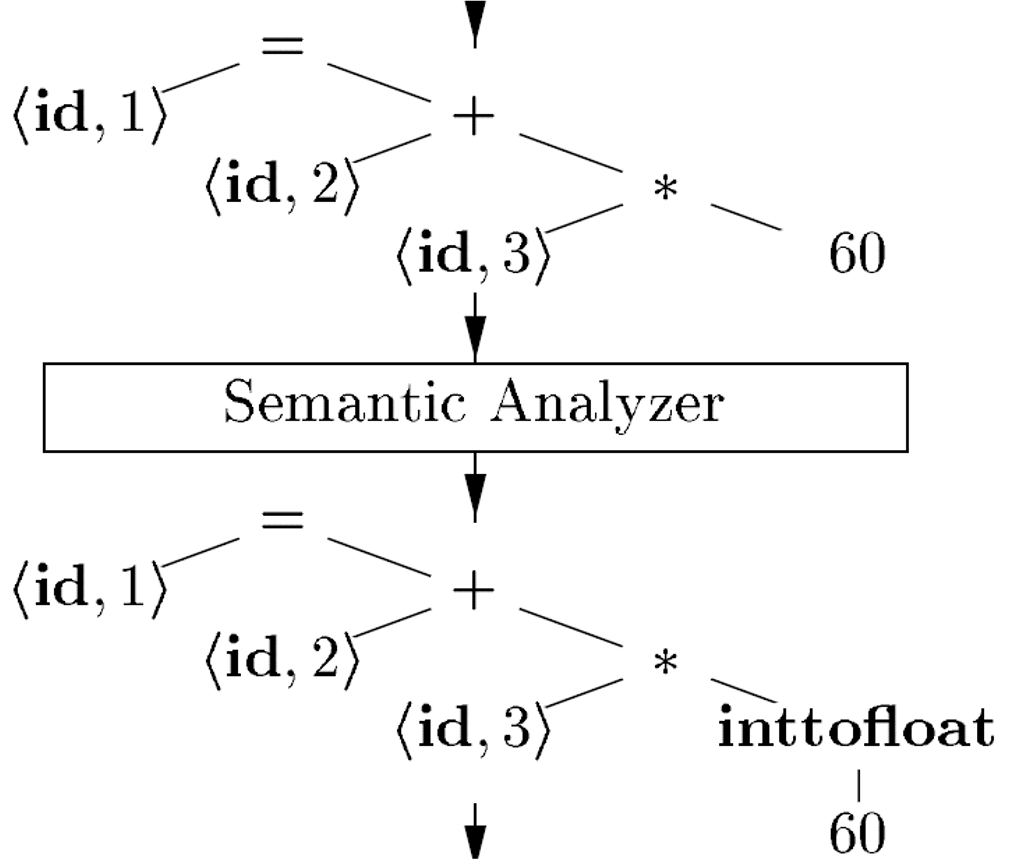
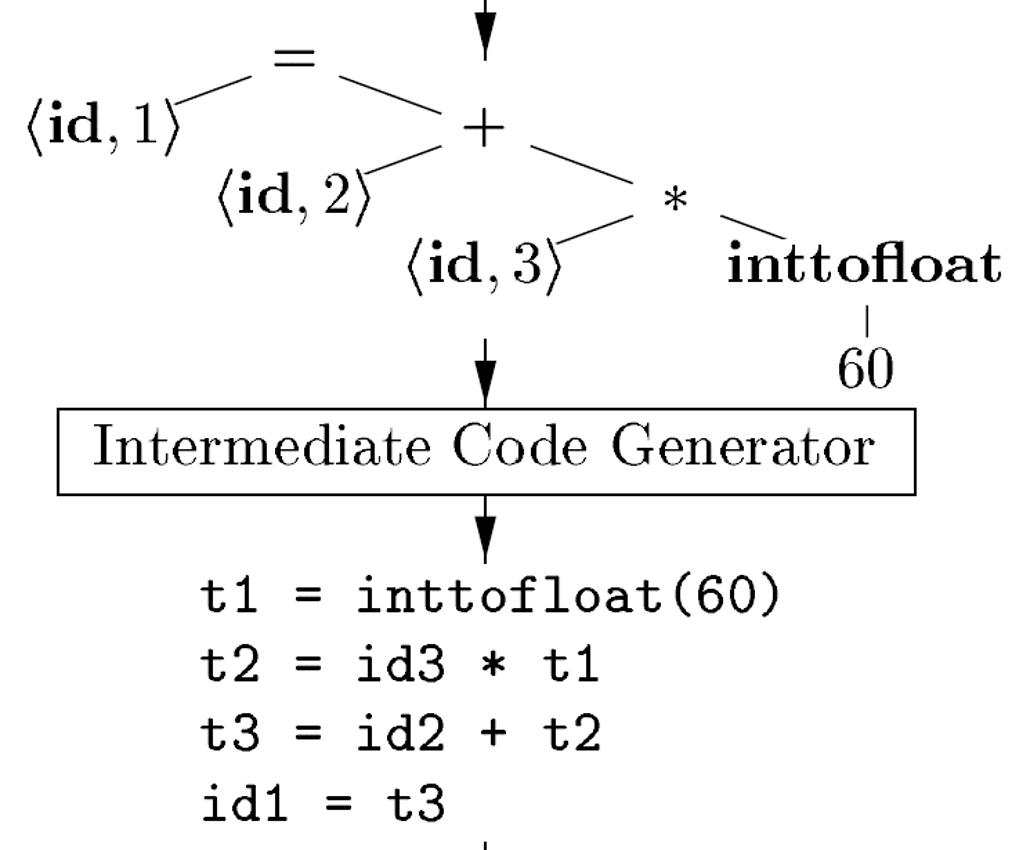
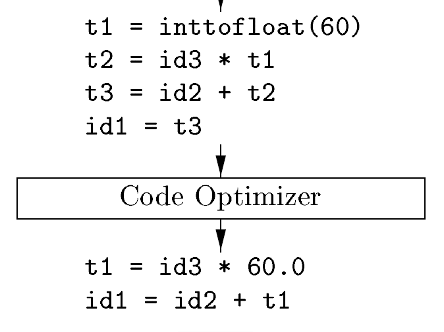
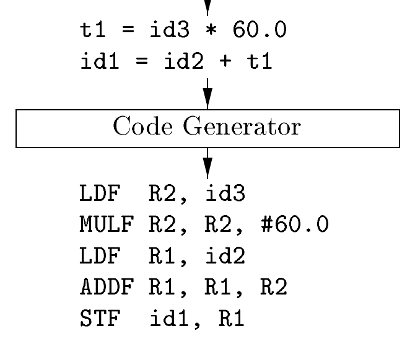